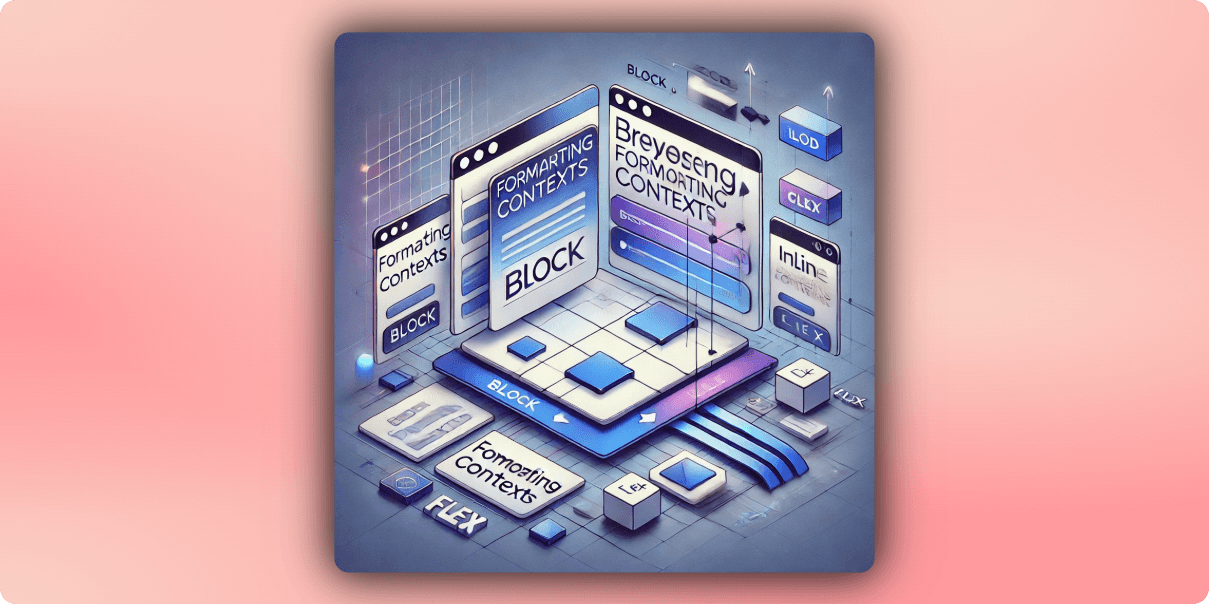
Hi there! 👋 Welcome to Frontend System Design — a series where we break down the core concepts and best practices for building robust frontend systems.
Understanding Browser Formatting Context
As frontend developers, we often encounter mysterious layout behaviors that seem to defy logic. Elements overflow their containers, margins collapse unexpectedly, or floats break layouts in ways that leave us scratching our heads.
Behind many of these frustrations lies a fundamental CSS concept that's rarely discussed in depth: formatting contexts. Understanding these invisible forces can transform how you approach layout challenges and eliminate the need for many hacky solutions.
The Problem With Traditional CSS Layout Solutions
Before diving into formatting contexts, let's acknowledge some common pain points:
- Float-based layouts that break and require clearfix hacks
- Margin collapsing creating unpredictable spacing
- Containing child elements that overflow their parents
- Text wrapping around floats when you don't want it to
- Elements sitting adjacent to floats with inconsistent spacing
Let's explore how formatting contexts can elegantly solve these problems without resorting to hacky workarounds.
What Are Formatting Contexts, Really?
A formatting context is essentially a layout environment - a defined area of the document with specific rules for how elements inside it are arranged and interact. Think of it as a "layout scope" that influences how its children behave.
Every element on your page participates in a formatting context. The magic happens when you explicitly create new formatting contexts to solve specific layout challenges.
Block Formatting Contexts: Multiple Creation Methods
While display: flow-root
is the most straightforward way to create a Block Formatting Context (BFC), there are several other methods, each with unique characteristics and potential side effects - ways to create a BFC?
Choosing the Right BFC Method
Your choice should depend on:
- Primary purpose: If creating a BFC is your only goal,
flow-root
is best - Layout needs: If you need specific layout behavior anyway (like flexbox), use that
- Content behavior: If content handling is important, consider
overflow
properties - Browser support: For older browsers,
overflow: auto
has better support thanflow-root
Block Formatting Contexts: Practical Use Cases
Problem 1: Containing Floated Elements
The Scenario: You have a section with a floated image and some text, but the section's background and border don't extend to contain the floated element.
<section class="card">
<img src="profile.jpg" class="avatar">
<p>This is a user profile card, but the floated image is causing the card's boundary to ignore it!</p>
</section>
.card {
border: 2px solid #3498db;
background-color: #f8f9fa;
padding: 16px;
}
.avatar {
float: left;
width: 80px;
margin-right: 16px;
}
The Problem: The floated image escapes the container's boundaries, as floats are taken out of normal flow.
The Solution: Create a new Block Formatting Context (BFC) in the container.
.card {
border: 2px solid #3498db;
background-color: #f8f9fa;
padding: 16px;
display: flow-root; /* Creates a new BFC */
}
Why It Works: A BFC contains all its elements, including floats. The display: flow-root
property creates a new BFC without side effects, making the container responsible for all its descendants.
Problem 2: Preventing Margin Collapse
The Scenario: You have two adjacent sections and want precise control over the spacing between them.
<section class="intro">
<h2>Introduction</h2>
<p>This section has a bottom margin of 30px.</p>
</section>
<section class="main-content">
<h2>Main Content</h2>
<p>This section has a top margin of 20px.</p>
</section>
.intro {
margin-bottom: 30px;
}
.main-content {
margin-top: 20px;
}
The Problem: Instead of getting 50px of space between sections (30px + 20px), you only get 30px because the margins collapse.
The Solution: Create a new BFC for one of the sections.
.main-content {
margin-top: 20px;
display: flow-root;
}
Why It Works: Margins only collapse within the same formatting context. By creating a new BFC, we prevent the margins from collapsing with elements outside this context.
Problem 3: Creating Side-by-Side Layouts Without Interference
The Scenario: You want a sidebar next to your main content, and you're using floats to achieve this layout.
<div class="layout">
<aside class="sidebar">
<h3>Categories</h3>
<ul>
<li>CSS Techniques</li>
<li>JavaScript</li>
<li>Performance</li>
</ul>
</aside>
<main class="content">
<h1>Understanding BFCs</h1>
<p>This text should appear beside the sidebar, not wrap under it...</p>
</main>
</div>
.sidebar {
float: left;
width: 200px;
}
.content {
/* Problem: text wraps around the floated sidebar */
}
The Problem: The text in .content
wraps around the floated sidebar instead of creating a clean column layout.
The Solution: Create a new BFC for the content area.
.content {
display: flow-root;
margin-left: 220px; /* Space for sidebar plus gap */
}
Why It Works: Elements in a new BFC don't wrap around floats outside their formatting context, creating a clean columnar layout.
Inline Formatting Contexts: The Subtle Details
Inline formatting contexts behave very differently from block contexts. Understanding them helps explain many confusing text layout behaviors.
The Scenario: You want to highlight some text in a paragraph with different styling.
<p>
Here is a paragraph with some <span class="highlight">important text</span>
that should stand out.
</p>
.highlight {
background-color: yellow;
padding: 10px;
margin: 10px;
border: 2px solid orange;
}
The Behavior: The horizontal padding, margin, and border work as expected, but vertical margin has no effect. The vertical padding and border appear but may overlap with text above and below.
Why This Happens: In an inline formatting context, vertical margins are ignored, and vertical padding and borders don't affect line height calculations, leading to potential overlap.
The Solution: If you need full box model behavior, convert the inline element to an inline-block:
.highlight {
background-color: yellow;
padding: 10px;
margin: 10px;
border: 2px solid orange;
display: inline-block;
}
Now the element participates in the inline formatting context (stays inline) but creates its own block formatting context internally, giving you the best of both worlds.
Flex Formatting Contexts: Layout Super Powers
When you create a flex container, you establish a flex formatting context for its children, unlocking powerful layout capabilities.
The Scenario: You need a card layout that adapts to different screen sizes, with consistent spacing and alignment regardless of content length.
Before Flexbox:
.card-container {
overflow: hidden; /* Clearfix hack */
}
.card {
float: left;
width: 30%;
margin-right: 3.33%;
margin-bottom: 20px;
}
.card:nth-child(3n) {
margin-right: 0;
}
/* Many more rules needed for proper alignment */
With Flex Formatting Context:
.card-container {
display: flex;
flex-wrap: wrap;
gap: 20px;
}
.card {
flex: 1 1 300px;
}
Why It's Better: The flex formatting context handles spacing, wrapping, and alignment automatically. Cards will grow and shrink based on available space, and the gap
property provides consistent spacing without margin collapse concerns.
Grid Formatting Contexts: Two-Dimensional Power
Grid formatting contexts take layout control even further by providing true two-dimensional layout capabilities.
The Scenario: You need a complex dashboard layout with areas of different sizes that must align perfectly across both rows and columns.
Before Grid:
.dashboard {
position: relative;
height: 100vh;
}
.header {
position: absolute;
top: 0;
left: 0;
right: 0;
height: 60px;
}
/* Many more absolute positioning rules... */
With Grid Formatting Context:
.dashboard {
display: grid;
grid-template-columns: 200px 1fr 300px;
grid-template-rows: auto 1fr auto;
grid-template-areas:
"header header header"
"sidebar main widgets"
"footer footer footer";
height: 100vh;
}
.header { grid-area: header; }
.sidebar { grid-area: sidebar; }
.main-content { grid-area: main; }
.widgets { grid-area: widgets; }
.footer { grid-area: footer; }
Why It's Better: The grid formatting context creates a comprehensive layout system where elements know their exact place in both dimensions, eliminating the need for complex positioning, floats, or negative margins.
Advanced: Combining Formatting Contexts
The real power comes when you strategically nest different formatting contexts to solve complex layout challenges.
The Scenario: You need a social media feed with floating profile pictures, flexible content areas, and perfectly aligned interaction buttons at the bottom of each post.
<div class="feed">
<article class="post">
<div class="post-header">
<img src="avatar.jpg" class="avatar">
<div class="user-info">
<h3>Username</h3>
<span class="timestamp">3 hours ago</span>
</div>
</div>
<div class="post-content">
<p>This is the post content with variable length.</p>
</div>
<div class="post-actions">
<button>Like</button>
<button>Comment</button>
<button>Share</button>
</div>
</article>
<!-- More posts -->
</div>
The Solution: A combination of formatting contexts.
.feed {
display: flex; /* Create a flex formatting context */
flex-direction: column;
gap: 16px;
}
.post {
display: flow-root; /* BFC to contain everything */
border: 1px solid #ddd;
border-radius: 4px;
}
.post-header {
display: flow-root; /* BFC to contain the float */
padding: 12px;
}
.avatar {
float: left;
width: 48px;
height: 48px;
border-radius: 50%;
margin-right: 12px;
}
.post-content {
padding: 0 12px;
}
.post-actions {
display: flex; /* Flex formatting context for the buttons */
justify-content: space-around;
padding: 8px 12px;
border-top: 1px solid #eee;
}
Why It Works: Each formatting context solves a specific layout challenge:
- The feed uses flex to stack posts with consistent spacing
- Each post creates a BFC to contain all its elements
- The post header creates a BFC to contain the floated avatar
- The post actions use flex to distribute buttons evenly
Performance Considerations
Creating new formatting contexts isn't just about layout control—it can also affect rendering performance:
Containment Benefits: New formatting contexts establish layout containment, meaning the browser can optimize rendering by treating them as isolated units.
Paint Boundaries: Many BFC-creating properties like
overflow
andtransform
also create paint boundaries, potentially improving performance for animations and scrolling.Reflow Scope: When content changes size, the reflow calculation might be limited to the formatting context rather than the entire page.
/* Performance optimization for a complex, frequently updating widget */
.stock-ticker {
display: flow-root;
contain: layout; /* Further enhances containment */
}
Debugging Tools: Identifying Formatting Contexts
Modern browser DevTools can help you identify formatting contexts:
Chrome/Edge: In the Elements panel, select "Layout" in the sidebar to see which elements establish formatting contexts.
Firefox: The "Layout" panel shows information about formatting contexts when you inspect elements.
Testing BFC behavior: You can quickly test if an element establishes a BFC by placing a float inside it and checking if the container expands to fit the float.
Conclusion: A New Way to Think About Layout
Understanding formatting contexts fundamentally changes how you approach CSS layout challenges. Instead of fighting against the browser with hacks and workarounds, you can work with its layout mechanisms by strategically creating the right formatting contexts.
The next time you face a layout challenge, ask yourself:
- "What formatting context is this element participating in?"
- "Would creating a new formatting context solve this problem?"
- "Which property should I use to create this formatting context with minimal side effects?"
This mental model will not only help you solve today's layout challenges but will continue to serve you well as CSS evolves with new layout mechanisms in the future.
Did you find this article helpful? Share it with your fellow developers on X or LinkedIn!
Quick Recap
- Formatting Contexts are layout environments that control how elements behave and interact with each other
- Block Formatting Contexts (BFCs) solve common layout issues like containing floats, preventing margin collapse, and creating clean column layouts
- Multiple ways to create BFCs include
display: flow-root
(recommended),overflow: auto/hidden
,display: flex/grid
, and others—each with different side effects - Inline Formatting Contexts handle text layout with specific rules (vertical margins don't apply)
- Flex and Grid Formatting Contexts provide powerful layout capabilities for modern designs
- Strategic combination of different formatting contexts can solve complex layout challenges elegantly
Coming up next: Browser Positioning System, Reflow, Composition Layers, and Browser Rendering—where we get into how browsers actually process and paint your pages. Stay tuned! 🚀